Войдите, чтобы подписаться
Подписчики
0
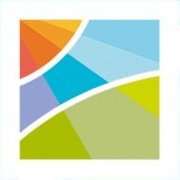
Небольшая модификация приложения Videos 3.3.6
Автор:
Zero108, в IPS Suite 4.x
-
Сейчас на странице 0 пользователей
Нет пользователей, просматривающих эту страницу.